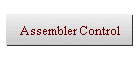
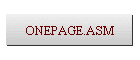
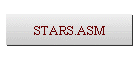
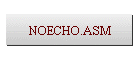
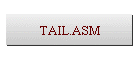
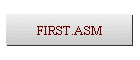
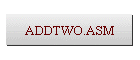
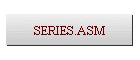
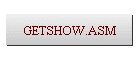
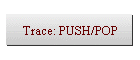
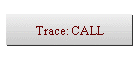
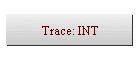
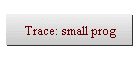
| |
Assembler Programming
This is an index page to resources to help you learn to program in Intel
Assembler and debug your programs using DOS DEBUG.
It contains the following sections:
Sample Programs
The sidebar contains links to many Intel assembler programs. First is
a one-page program named onepage.asm that you can
select and download to make sure your assembler and linker are working
correctly. (If you can't assemble and run this simple program without
any warnings or errors, something is wrong with your assembler or linker!)
The command lines used to assemble and link this program are given in the
comments at the beginning of the program. There is also a Web page describing
them. Either put the ASM and VAL programs in the same directory as your
program, or adjust your DOS PATH variable to include where they reside.
Read the documentation for more details on how the ASM and VAL programs work.
Next is Alan's stars.asm program that prints
numbers of asterisks based on one key of input, and noecho.asm
that loops reading characters without echoing them to the screen.
The more complex tail.asm program displays the
Command Tail in the Program Segment Prefix. It shows how a set of
high-level language statements (e.g. in C language) might be turned into
assembler by a compiler (though this program was translated by hand, not by a
compiler).
Next is a longer program (mostly comments!) named first.asm
that uses Alan
Pinck's I/O Package. (This package contains some subroutines for
inputting and outputting numbers.) You will need Alan's I/O package in
the current directory on your disk to assemble this example. This
program is a good test to see if the I/O package downloaded to your computer
correctly. Program addtwo.asm is another
example that uses the I/O package.
The program series.asm also uses Alan's I/O
package and is a fairly direct translation of LMC (Little-Man Computer)
mnemonic instructions into Intel mnemonic instructions. The original web
page describing this algorithm is here: http://elearning.algonquincollege.com/coursemat/pincka/dat2343/lect054.htm
Program Control Flow
Assembler has no high-level control flow statements. To achieve the
same effect, you must use conditional branching. See the Assembler Control page for details.
Programs submitted for marking in this course get maximum marks if they
adhere to the following structure:
Text editing your ASM Programs
The ASM and MASM assembler programs both insist that files be well-formed,
with proper line end characters on every line, including the last line of the
file. You cannot create a text file using a word processor, unless
you explicitly select "Save as text" when you save the
file. If you use Windows Notepad to build an ASM file, make sure
that the last line of the file ends in a RETURN character. Failure to do
this will result in "premature end of file" errors of this type:
C:> asm /s onepage ;
End of file encountered on input file
end start
onepage.asm(35): ERROR#85 - Premature end of file
Edit the file to add the missing RETURN on the last line of the file.
Reading the file into DOS EDIT and writing it out will fix the problem, as
will reading the file into VIM (an open source version of Unix VI) and writing
it out again.
When in doubt, put a few blank lines at the end of your program source
files!
If you see programming as a career, you will do well to pick and learn a
proper text editor that has cut buffers, key macros, and global
search-and-replace capabilities. (I use VIM on both Unix and
Windows.) Keyboard and editing skills should not stand in your way of a
good job!
The course makes extensive use of the DOS DEBUG command.
Here are some links to descriptions of how to use the DOS DEBUG command:
http://chesworth.com/pv/technical/dos_debug_tutorial.htm
http://www.datainstitute.com/debug1.htm
Saving output from DEBUG
Some of the assignments require you to save the output of DEBUG in a
file. If you're running in a DOS Window under Windows 9x, you can always
use the mouse to copy text and paste it into another application such as
Notepad, Wordpad, or Write. (Do not save the window as a graphic
using Print Screen - the resulting file is huge and unnecessary. Save
the text only, using cut-and-paste into another application such as
Write, Wordpad, or Notepad, and print from there.)
When you print some screen text or programs, you must use a Courier or Terminal fixed-width font so
that the text lines up. Do not print with a variable-width font
such as Times, Tahoma, or Arial!
If you're running in pure-DOS mode, without Windows, study the examples
below under the heading DEBUG Scripts.
You can enter a few DEBUG commands into a text file, and have DEBUG read the
file and execute the commands while you redirect the output into an output
file. Then, you can print the output files (using a Courier
or Terminal fixed-width font).
The PUSH, POP, CALL, and INT instructions all use the stack (the memory
area pointed at by SS:SP). The following DEBUG scripts and their
annotated output files show what happens. Each of the input files was
run through DEBUG to produce the corresponding output file, to which
explanatory comments were added by hand:
C:> debug <push_pop.txt >push_pop_out.txt
C:> debug <call_push.txt >call_push_out.txt
C:> debug <int_push.txt >int_push_out.txt
Read these Output files for annotated examples of the workings of PUSH/POP,
CALL, and INT:
Homework, test, and exam questions typically show one of these types of
instructions and ask you to describe which registers and what memory is
affected after the instruction executes (see the Homework questions!).
The DOS DEBUG command lets you single-step through your programs to debug
them. You can also use it to load raw disk sectors from disks and examine
them (as done in Project 4).
One of the handy things you can do with DEBUG is prepare small text files
of DEBUG commands (and even include assembler instructions) and run them
through DEBUG using command line redirection to get a quick idea of how
something works:
C:> debug <inputcommands >outputfile
See the script file debug_script.txt
and its corresponding output file debug_script_out.txt. I've edited the output
to add comments explaining what I was doing in the script file, and how to
create the script file.
If you're trying to dump disk sectors (as you do in Project 4 in this
course), you may find these kinds of DEBUG scripts, used with command line
redirection, necessary for saving your DEBUG output into files for later
printing: Script file: debug_dump.txt
Script output: debug_dump_out.txt
Tracing COM file programs
DEBUG is able to load and let you single-step through ".COM"
format executables. The quickest way to get the executable loaded into
DEBUG is to give the program you wish to debug as a command argument.
All .COM programs start at segment offset 0100h, so that's where you'll find
your first instructions, ready to be traced:
C:> debug myprog.com
-u 0100
1454:0100 BE8100 MOV SI,0081
1454:0103 803C0D CMP BYTE PTR [SI],0D
1454:0106 7421 JZ 0129
1454:0108 803C20 CMP BYTE PTR [SI],20
1454:010B 7511 JNZ 011E
1454:010D BA2F01 MOV DX,012F
1454:0110 B409 MOV AH,09
1454:0112 CD21 INT 21
1454:0114 83C601 ADD SI,+01
1454:0117 803C20 CMP BYTE PTR [SI],20
1454:011A 74F8 JZ 0114
1454:011C EBE5 JMP 0103
1454:011E 8A14 MOV DL,[SI]
-
With your program in memory, you are now ready to debug it by
single-steping through it using the "Trace" or "Proceed"
commands, watching the flow of control and the values of the registers.
If you want to supply command line arguments to a program being debugged,
simply add them to your DOS command line when you call DEBUG:
C:> debug myprog.com argument1 argument2 arg3 ...etc...
Using "Trace" vs. "Proceed" in DEBUG
Don't use "Trace" to trace an INT instruction; use
"Proceed" instead. If you trace the INT instruction,
you will end up tracing the call to the interrupt service routine in DOS that
actually performs the interrupt service, and that can be many hundreds or
thousands of instructions! The "Proceed" instruction will not
trace the interrupt service routine; it will simply let it execute (without
tracing each instruction) and it will pause when the service routine returns
to your program.
|